Functional programming in javascript: Function composition
Function composition is one the key features (among others) of functional programming. Programming languages that offer higher order functions as a feature can potentially use function composition. But, still, programmers need to be aware of some key concepts to successfully apply this pattern in our code.
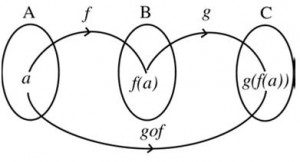
Function composition, as defined on Wikipedia, is an act or mechanism to combine simple functions to build more complicated ones. In other words, we can define new functions, equivalent to the result of chaining a set of given functions, so the input of function i is the output (or result) of function i-1. Let’s see an example in javascript.
Having these two functions:
function double(x) {
return x*2;
}
function triple(x) {
return x*3;
}
We define sixtimes as the composition of the two previous:
function sixtimes(x) {
return double(triple(x));
}
Obviously in this case it would have been easier just to multiply x by 6 inside sixtimes, but let me continue with this dumb example just to get to the concept.
This pattern is going to be used widespread in functional programming, so one could define a compose function that would return a new function resulting of the composition of two given:
function compose(f, g) {
return function(x) {
return g(f(x));
}
}
Now, using compose to define our previous function sixtimes, would be as easy as:
var sixtimes = compose(triple, double);
Isn’t it beautiful?
In following posts I will show you some gems extracted from Javascript Allongé by Reginald Braithwaite, where the author explains some useful composition tips and a really nice concept he calls Function decorators. (Update: after digging a little bit, I found this concept comes from Python world, where the very syntax allows you to list decorators for functions in its definition)
Until then you’re welcome to take a look at my presentation slides and code I used during a talk in a Ruby local users group (valencia.rb) comparing OOP and FP: